Spring Boot 单元测试 MockMvc 模拟 HTTP 请求
Spring Boot 单元测试 HTTP About 4,132 wordsMockMvc
@WebMvcTest
标注的单元测试类中,可以注入MockMvc
对象。
@SpringBootTest
标注的单元测试类,还需要加@AutoConfigureMockMvc
注解。
MockMvc
模拟发送HTTP
请求。
GET 请求
andExpect
:期望的结果
andDo
:打印结果
andReturn
:返回最终的结果
content().string()
:判断返回字符串中的值。
mockMvc.perform(MockMvcRequestBuilders.get("/hi")
.header("token", "test-token")
.param("username", "test-username")
)
.andExpect(MockMvcResultMatchers.status().isOk())
.andDo(MockMvcResultHandlers.print())
.andExpect(MockMvcResultMatchers.content().string(containsString("msg")))
.andReturn();
POST 请求
jsonPath()
:提取JSON
字段进行判断。
mockMvc.perform(MockMvcRequestBuilders.post("/hi")
.contentType(MediaType.APPLICATION_JSON)
.content("{\"code\":\"ddd-d\",\"name\":\"this is name\"}")
)
.andExpect(MockMvcResultMatchers.status().isOk())
.andDo(MockMvcResultHandlers.print())
.andExpect(MockMvcResultMatchers.jsonPath("code").value(0))
.andReturn();
示例代码
@WebMvcTest
import static org.hamcrest.Matchers.containsString;
@WebMvcTest(HiController.class)
class HiControllerTests {
@Autowired
MockMvc mockMvc;
@MockBean
HelloService helloService;
@Test
@DisplayName("测试 GetMapping 接口")
void testGetMappingWithMockMvc() throws Exception {
BDDMockito.given(helloService.sayHello(1))
.willReturn(Response.builder().msg("response msg").build());
mockMvc.perform(MockMvcRequestBuilders.get("/hi")
.header("token", "test-token")
.param("username", "test-username")
)
.andExpect(MockMvcResultMatchers.status().isOk())
.andDo(MockMvcResultHandlers.print())
.andExpect(MockMvcResultMatchers.content().string(containsString("msg")))
.andReturn();
}
@Test
@DisplayName("测试 PostMapping 接口")
void testPostMappingWithMockMvc() throws Exception {
mockMvc.perform(MockMvcRequestBuilders.post("/hi")
.contentType(MediaType.APPLICATION_JSON)
.content("{\"code\":\"ddd-d\",\"name\":\"this is name\"}")
)
.andExpect(MockMvcResultMatchers.status().isOk())
.andDo(MockMvcResultHandlers.print())
.andExpect(MockMvcResultMatchers.jsonPath("code").value(0))
.andReturn();
}
}
@AutoConfigureMockMvc
@SpringBootTest(classes = {SpringBootTestApplication.class}, webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
@AutoConfigureMockMvc
class HelloControllerTests {
@Autowired
MockMvc mockMvc;
// @MockBean
// HelloService helloService;
@MockBean
UserMapper userMapper;
@Test
@DisplayName("测试 GetMapping 接口")
void testGetMappingWithMockMvc() throws Exception {
BDDMockito.given(userMapper.getUserById(1))
.willReturn(new User(1,"张三","zhangsan"));
mockMvc.perform(MockMvcRequestBuilders.get("/hello")
.header("token", "test-token")
.param("username", "test-username")
)
.andExpect(MockMvcResultMatchers.status().isOk())
.andDo(MockMvcResultHandlers.print())
.andExpect(MockMvcResultMatchers.content().string(containsString("zhangsan")))
.andReturn();
}
@Test
@DisplayName("测试 PostMapping 接口")
void testPostMappingWithMockMvc() throws Exception {
mockMvc.perform(MockMvcRequestBuilders.post("/hello")
.contentType(MediaType.APPLICATION_JSON)
.content("{\"code\":\"ddd-d\",\"name\":\"this is name\"}")
)
.andExpect(MockMvcResultMatchers.status().isOk())
.andDo(MockMvcResultHandlers.print())
.andExpect(MockMvcResultMatchers.jsonPath("code").value(0))
.andReturn();
}
}
参考
Views: 1,830 · Posted: 2022-10-17
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
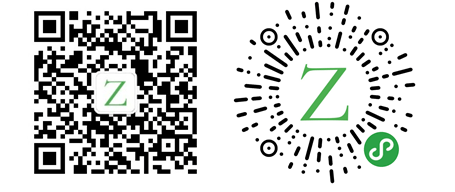
Loading...