Vue provide inject 组件传值
Vue About 2,270 words作用
祖先组件向其所有子孙后代注入一个依赖(就是传递参数)
祖先组件
provide
:提供数据
<template>
<div>
<p>
Count from Root: {{ count }}
</p>
<p>
Price from Root: {{ info }}
</p>
<button @click="addRootCount">Add Root Count</button>
<ChildComponent></ChildComponent>
</div>
</template>
<script>
import ChildComponent from "@/components/project-inject/ChildComponent";
export default {
name: "RootComponent",
components: {ChildComponent},
data() {
return {
message: "Hello Root Component",
count: 0,
info: {
price: 20
}
}
},
methods: {
addRootCount() {
this.count++;
this.info.price++;
}
},
provide() {
console.log("root provide")
return {
message: this.message,
count: this.count,
info: this.info
}
},
}
</script>
子孙组件
inject
:接受数据。
可以是数组类型,也可以是对象类型。
数组类型:对应的字符串元素必须是provide
传递的名字。
对象类型:可以自定义名称,使用from
表示provide
传递的名字。
<template>
<div>
<p>
This is Deep Child
</p>
<p>
Deep Child Inject count: {{ deepCount }}
</p>
<p>
Deep Child Inject price: {{ deepInfo }}
</p>
</div>
</template>
<script>
export default {
name: "DeepChildComponent",
data() {
return {
deepCount: this.customCount,
deepInfo: this.customInfo,
}
},
inject: {
"customCount": {
from: "count",
default: 100
},
"customInfo": {
from: "info",
default: ()=>{}
}
},
}
</script>
响应式
provide
和inject
绑定的数据不是可响应的,需要使用可监听对象才能实现响应。
生命周期
root provide
root created
deep child created
deep child mounted
root mounted
说明
如果数据在data
中定义为null
,在created
或mounted
阶段赋值为对象,则也不能实现数据的响应式传递。
祖先组件(此例子是不能实现数据的响应式传递):
export default {
name: "RootComponent",
components: {ChildComponent},
data() {
return {
info: null
}
},
methods: {
addRootCount() {
this.count++;
this.info.price++;
}
},
provide() {
return {
info: this.info
}
},
created() {
this.info = {
price: 50
}
},
}
参考
V2
https://cn.vuejs.org/v2/api/index.html#provide-inject
V3
Views: 950 · Posted: 2023-01-06
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
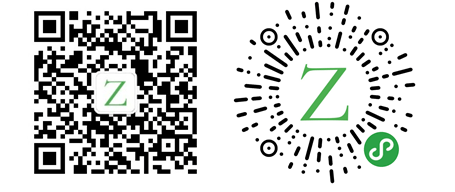
Loading...