Java 8 Stream 中的 group by 分组
Java About 2,134 words需求
使用Collectors
的groupingBy
完成各种分组操作。
Bean 类
@Data
class Student {
private String name;
private int score;
private int age;
}
集合
Student zs1 = new Student("zs", 100, 20);
Student ls = new Student("ls", 90, 25);
Student ww = new Student("ww", 90, 23);
Student zs2 = new Student("zs", 85, 30);
List<Student> list = List.of(zs1, ls, ww, zs2);
学生姓名分组
Map<String, List<Student>> nameStudentsMap = list.stream().collect(Collectors.groupingBy(Student::getName));
输出
{ww=[Student{name='ww', score=90, age=23}], ls=[Student{name='ls', score=90, age=25}], zs=[Student{name='zs', score=100, age=20}, Student{name='zs', score=85, age=30}]}
按分数分组取学生对象集合
Map<Integer, List<Student>> scoreStudentsMap = list.stream().collect(Collectors.groupingBy(Student::getScore));
输出:可以看到90
分有2
个学生,其余都是1
个学生。
{100=[Student{name='zs', score=100, age=20}], 85=[Student{name='zs', score=85, age=30}], 90=[Student{name='ls', score=90, age=25}, Student{name='ww', score=90, age=23}]}
统计分组中包含的个数
Map<Integer, Long> scoreCountMap = list.stream().collect(Collectors.groupingBy(Student::getScore, Collectors.counting()));
输出:可以看到90
分有2
个人,其余都是1
个人。
{100=1, 85=1, 90=2}
按分数分组取学生姓名集合
Collectors.groupingBy
分组中使用Collectors
的mapping
映射学生到姓名的关系。
Map<Integer, List<String>> scoreStudentNamesMap = list.stream().collect(Collectors.groupingBy(Student::getScore, Collectors.mapping(Student::getName, Collectors.toList())));
输出
{100=[zs], 85=[zs], 90=[ls, ww]}
按分数分组取学生年龄大于 20 的学生集合
Map<Integer, Set<Student>> scoreStudentsAgeGt20Map = list.stream().collect(Collectors.groupingBy(Student::getScore, Collectors.filtering(student -> student.getAge() > 20, Collectors.toSet())));
输出
{100=[], 85=[Student{name='zs', score=85, age=30}], 90=[Student{name='ww', score=90, age=23}, Student{name='ls', score=90, age=25}]}
按分数分组取学生姓名以 z 开始的姓名集合
Map<Integer, Set<String>> scoreStudentNameStartWithMap = list.stream().collect(Collectors.groupingBy(Student::getScore, Collectors.mapping(Student::getName, Collectors.filtering(name -> name.startsWith("z"), Collectors.toSet()))));
输出
{100=[zs], 85=[zs], 90=[]}
Views: 865 · Posted: 2023-06-09
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
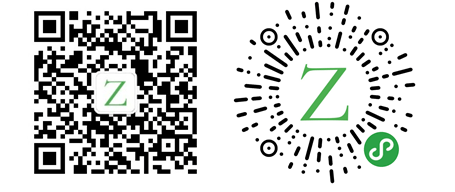
Loading...