设计模式之命令模式
设计模式 Java About 3,283 words作用
将一个请求封装为一个对象,从而使你可以用不同的请求对客户进行参数化,对请求排队和记录请求日志,以及支持可撤销的操作。
原理
Invoker:调用者角色。
Command:命令角色,需要执行的所有命令都在这里,可以是接口或抽象类。
Receiver:接受者角色,知道如何实施和执行一个请求相关的操作。
ConcreteCommand:将一个接受者对象与一个动作绑定,调用接受者相应的操作,实现 execute。
案例
Invoker调用者
public class RemoteController {
private Command[] onCommands;
private Command[] offCommands;
private Command undoCommand;
public RemoteController() {
onCommands = new Command[5];
offCommands = new Command[5];
for (int i = 0; i < 5; i++) {
onCommands[i] = new NoCommand();
offCommands[i] = new NoCommand();
}
}
public void setCommand(int index, Command onCommand, Command offCommand) {
this.onCommands[index] = onCommand;
this.offCommands[index] = offCommand;
}
public void onButtonPushed(int index) {
onCommands[index].execute();
undoCommand = onCommands[index];
}
public void offButtonPushed(int index) {
offCommands[index].execute();
undoCommand = offCommands[index];
}
public void undoButtonPushed() {
undoCommand.undo();
}
}
Command命令
public interface Command {
void execute();
void undo();
}
Receiver
public class LightReceiver {
public void on() {
System.out.println("电灯打开了");
}
public void off() {
System.out.println("电灯关闭了");
}
}
ConcreteCommand
public class LightOnCommand implements Command {
private LightReceiver lightReceiver;
public LightOnCommand(LightReceiver lightReceiver) {
this.lightReceiver = lightReceiver;
}
@Override
public void execute() {
lightReceiver.on();
}
@Override
public void undo() {
lightReceiver.off();
}
}
public class LightOffCommand implements Command {
private LightReceiver lightReceiver;
public LightOffCommand(LightReceiver lightReceiver) {
this.lightReceiver = lightReceiver;
}
@Override
public void execute() {
lightReceiver.off();
}
@Override
public void undo() {
lightReceiver.on();
}
}
public class NoCommand implements Command {
@Override
public void execute() {
}
@Override
public void undo() {
}
}
调用
public class Client {
public static void main(String[] args) {
LightReceiver lightReceiver = new LightReceiver();
LightOnCommand lightOnCommand = new LightOnCommand(lightReceiver);
LightOffCommand lightOffCommand = new LightOffCommand(lightReceiver);
RemoteController remoteController = new RemoteController();
remoteController.setCommand(0, lightOnCommand, lightOffCommand);
remoteController.onButtonPushed(0);
remoteController.undoButtonPushed();
System.out.println("--------------------");
remoteController.offButtonPushed(0);
remoteController.undoButtonPushed();
}
}
源码
org.springframework.jdbc.core.JdbcTemplate
Invoker:org.springframework.jdbc.core.JdbcTemplate#execute(org.springframework.jdbc.core.StatementCallback
Command:org.springframework.jdbc.core.StatementCallback
ConcreteCommand:org.springframework.jdbc.core.JdbcTemplate#query(java.lang.String, org.springframework.jdbc.core.ResultSetExtractor
Views: 2,145 · Posted: 2019-12-26
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
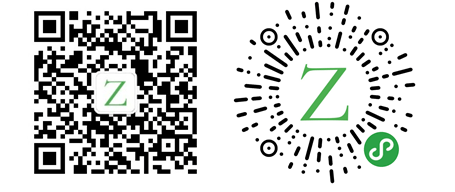
Loading...