Spring Boot 使用 JavaMailSender 发送邮件
Spring Boot Java About 2,696 words添加依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
添加配置
配置用户名、密码、邮件服务host
和端口。
spring:
mail:
username: 123@test.com
host: smtp.test.com
password: testpwd
port: 25
发送简易邮件
设置发送账号、接收账号、抄送账号、密送账号。
@SpringBootApplication
public class DemoApplication implements ApplicationRunner {
@Resource
private JavaMailSender javaMailSender;
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
@Override
public void run(ApplicationArguments args) throws Exception {
//建立邮件消息
SimpleMailMessage mainMessage = new SimpleMailMessage();
//发送方
mainMessage.setFrom("123@test.com");
//接收方
mainMessage.setTo("456@test.com");
//抄送
mainMessage.setCc("789@test.com");
//密送
mainMessage.setBcc("111@test.com");
//发送的标题
mainMessage.setSubject("主题2");
//发送的内容
mainMessage.setText("内容2");
javaMailSender.send(mainMessage);
}
}
发送复杂邮件
带附件、富文本邮件。
注意:附件尽可能选择使用
DataSource
而非File
。富文本使用img
标签时需有占位符cid:xxx
,其中cid
是固定格式,xxx
是自定义标识,在addInline
方法中设置xxx
标识对应的流。
MimeMessage mimeMessage = javaMailSender.createMimeMessage();
MimeMessageHelper mimeMessageHelper = new MimeMessageHelper(mimeMessage, true);
mimeMessageHelper.setFrom("zhangbj94@chinaunicom.cn");
mimeMessageHelper.setTo("zhangbj94@chinaunicom.cn");
mimeMessageHelper.setSubject("主题3333");
ClassPathResource classPathResource = new ClassPathResource("application.yml");
File attachmentFile = classPathResource.getFile();
mimeMessageHelper.addAttachment(MimeUtility.encodeWord("附件1:application.yml"), attachmentFile);
ByteArrayDataSource attachmentDataSource = new ByteArrayDataSource(classPathResource.getInputStream(), "application/octet-stream");
mimeMessageHelper.addAttachment(MimeUtility.encodeText("附件2:application.yml"), attachmentDataSource);
//富文本
mimeMessageHelper.setText("<html><body><p>你好:</p><div><img src='cid:contentId1'></div><a href='https://www.baidu.com'>https://www.baidu.com</a></body></html>",true);
ClassPathResource classPathResource2 = new ClassPathResource("pic/test.png");
String type = Files.probeContentType(Paths.get(classPathResource2.getURI()));
ByteArrayDataSource inlineDataSource = new ByteArrayDataSource(classPathResource2.getInputStream(), type);
mimeMessageHelper.addInline("contentId1", inlineDataSource);
javaMailSender.send(mimeMessage);
完整代码
https://github.com/fendoudebb/learning/tree/master/java/learn-spring-boot/mail-sender
Views: 3,577 · Posted: 2020-05-21
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
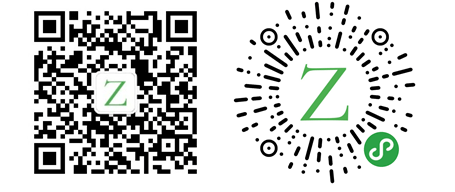
Loading...