Angular 相关知识点
Angular About 2,638 words示例
<div *ngFor="let product of products">
<h3>
<a [title]="product.name + ' details'">
{{ product.name }}
</a>
</h3>
<p *ngIf="product.description">
Description: {{ product.description }}
</p>
<button (click)="share()">
Share
</button>
</div>
结构型指令
*ngFor
*ngFor
类似for
循环,遍历生成子节点。
示例中h3
标签及其子标签会被生成多个。
*ngIf
*ngFor
只有当表达式中的字段值不为空时才渲染。
示例中p
标签只有当product
实例的description
属性不为空时才生成。
插值语法
{{ }}
可以把属性值渲染为文本。
示例中会渲染出product
的name
属性。
属性绑定语法
[ ]
可以在模板表达式中使用属性值。通俗讲就是将标签的属性使用[]
括起来,可以在等于号后字符串中使用变量及字符串拼接。
示例中a
标签的title
属性就等于product
的name
加上 details
字符串。
事件绑定
( )
可以讲事件绑定在指定标签上。
示例中button
绑定了click
点击事件,会触发xxx.component.ts
中定义的share()
方法。
组件间通讯
父组件传递给子组件
父组件通过[ ]
将自己component
中的属性传递给子组件。
<div>
<app-product-alerts
[product]="product">
</app-product-alerts>
</div>
子组件component
,使用@Input
接收父组件输入的对象。
import {Input} from '@angular/core';
import {Product} from "../products";
export class ProductAlertsComponent {
@Input()
product!: Product;
}
子组件HTML
可以获取子组件component
中接收到的属性。
<p *ngIf="product && product.price > 700">
<span>Hello</span>
</p>
子组件传递给父组件
子组件component
中使用@Output
注解标注notify
成员变量(变量名可随意取)。
export class ProductAlertsComponent {
@Output()
notify = new EventEmitter();
}
子组件HTML
通过点击事件发送通知事件。(notify
是component
中定义的成员变量名称,emit()
方法是EventEmitter
类的方法)
<p>
<button (click)="notify.emit()">Notify Me</button>
</p>
父组件HTML
中在子组件标签中使用( )
接收notify
事件,并触发component
中定义的onNotify()
方法。
( )
中的notify
为子组件中定义的@Output
标注的变量名(变量名可随意取)。
<div>
<app-product-alerts
(notify)="onNotify()">
</app-product-alerts>
</div>
父组件component
中定义回调方法(方法名可随意取,需对应HTML
中的方法)。
export class ProductListComponent {
onNotify() {
window.alert("onNotifyxxx");
}
}
创建组件和服务
创建组件
在项目根目录执行,会自动在src/app
目录下创建product-details
文件夹,并生成ts
、html
、css
、spec.ts
四个文件。
ng generate component product-alerts
输出
D:\Demo>ng generate component product-details
CREATE src/app/product-details/product-details.component.html (30 bytes)
CREATE src/app/product-details/product-details.component.spec.ts (683 bytes)
CREATE src/app/product-details/product-details.component.ts (310 bytes)
CREATE src/app/product-details/product-details.component.css (0 bytes)
UPDATE src/app/app.module.ts (1149 bytes)
创建服务
并不会创建cart
文件夹。而是直接创建ts
、spec.ts
两个文件。
ng generate service cart
输出
D:\Demo>ng generate service cart
CREATE src/app/cart.service.spec.ts (347 bytes)
CREATE src/app/cart.service.ts (133 bytes)
启动服务
指定端口
ng serve --port=4200
指定域名
ng serve --host=test.com
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
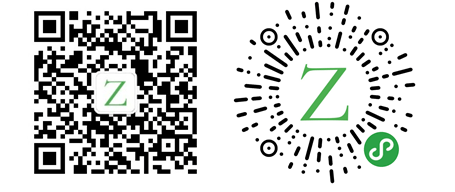