Spring OpenFegin 实现微服务间 RPC 调用
OpenFeign Spring Boot About 3,517 words添加依赖
Spring Boot 2.6.7
、openfeign 3.1.2
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.7</version>
<relativePath/>
</parent>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
<version>3.1.2</version>
</dependency>
第三方服务
@RestController
public class HelloController {
@GetMapping("/hello/{id}")
public Response helloGetPathVariable(@PathVariable("id") Integer id) throws InterruptedException {
// Thread.sleep(4000);
return Response.builder().msg("ok-get-PathVariable-" + id).build();
}
}
启用 Feign
使用@EnableFeignClients
开启FeginClient
。
@EnableFeignClients
@SpringBootApplication
public class LearnSpringCloudOpenfeignApplication {
public static void main(String[] args) {
SpringApplication.run(LearnSpringCloudOpenfeignApplication.class, args);
}
}
FeginClient
使用@FeignClient
表示改类是一个Fegin
客户端。
@FeignClient(name = "my-feign-client", url = "http://localhost:8080")
public interface MyFeignService {
@GetMapping("/hello/{id}")
Response helloGetPathVariable(@PathVariable("id") Integer id);
}
RequestInterceptor
添加请求拦截器,需在application.yml
中配置
@Slf4j
public class TokenRequestInterceptor implements RequestInterceptor {
@Override
public void apply(RequestTemplate requestTemplate) {
ServletRequestAttributes attributes = (ServletRequestAttributes) RequestContextHolder.getRequestAttributes();
HttpServletRequest request = attributes.getRequest();
String token = request.getHeader("token");
log.info("token request interceptor#{}, token#{}", requestTemplate, token);
}
}
Fegin 配置文件
compression
:开启请求和响应的压缩default
:默认Fegin
客户端配置loggerLevel
:日志等级(需配合logging.level
)logging.level
:需同时开启feign
的loggerLevel
requestInterceptors
:请求拦截器,责任链
feign:
compression:
request:
enabled: true
mime-types: text/xml,application/xml,application/json
min-request-size: 2048
response:
enabled: true
client:
config:
default:
connect-timeout: 5000
read-timeout: 3000
loggerLevel: full
requestInterceptors:
- com.example.learnspringcloudopenfeign.feign.interceptor.TokenRequestInterceptor
- com.example.learnspringcloudopenfeign.feign.interceptor.TestRequestInterceptor
logging:
level:
com.example.learnspringcloudopenfeign: debug
可能出现的错误
Description:
Your project setup is incompatible with our requirements due to following reasons:
- Spring Boot [2.6.7] is not compatible with this Spring Cloud release train
Action:
Consider applying the following actions:
- Change Spring Boot version to one of the following versions [2.4.x, 2.5.x] .
You can find the latest Spring Boot versions here [https://spring.io/projects/spring-boot#learn].
If you want to learn more about the Spring Cloud Release train compatibility, you can visit this page [https://spring.io/projects/spring-cloud#overview] and check the [Release Trains] section.
If you want to disable this check, just set the property [spring.cloud.compatibility-verifier.enabled=false]
原因
解决办法
方法一
升级openfeign
版本。
方法二
禁用兼容性校验。
spring.cloud.compatibility-verifier.enabled=false
openfeign 版本信息
https://spring.io/projects/spring-cloud-openfeign#learn
帮助文档
https://docs.spring.io/spring-cloud-openfeign/docs/current/reference/html
Views: 1,860 · Posted: 2022-10-05
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
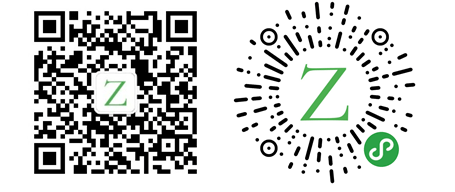
Loading...