Spring Boot 单元测试 @WebMvcTest 对 Controller 进行单独测试
Spring Boot 单元测试 About 2,352 words需求
只能Controller
中的逻辑进行单元测试,Service
层不进行测试。
@MockBean
使用@MockBean
模拟Service
层,给定Service
层返回,人为控制Service
层的返回值。
@WebMvcTest
需要单元测试的Controller
类。指定一个需要测试的Controller
类。
Controller 类
@Slf4j
@RestController
public class HiController {
@Resource
private HelloService helloService;
@GetMapping("/hi")
public Response hiGet(@RequestHeader("token") String token, @RequestParam("username") String username, HttpServletRequest request) {
log.info("hello token#{}, username#{}, request#{}", token, username, request);
Response response = helloService.sayHello(1);
return response;
}
@PostMapping("/hi")
public Response hiPost(@Valid @RequestBody HelloRequest request) {
log.info("helloPost request#{}", request);
return Response.builder().msg(request.getCode()).build();
}
}
单元测试类
@WebMvcTest(HiController.class)
class HiControllerTests {
@Autowired
MockMvc mockMvc;
@MockBean
HelloService helloService;
@Test
@DisplayName("测试 GetMapping 接口")
void testGetMappingWithMockMvc() throws Exception {
BDDMockito.given(helloService.sayHello(1))
.willReturn(Response.builder().msg("response msg").build());
mockMvc.perform(MockMvcRequestBuilders.get("/hi")
.header("token", "test-token")
.param("username", "test-username")
)
.andExpect(MockMvcResultMatchers.status().isOk())
.andDo(MockMvcResultHandlers.print())
.andExpect(MockMvcResultMatchers.content().string(containsString("msg")))
.andReturn();
}
@Test
@DisplayName("测试 PostMapping 接口")
void testPostMappingWithMockMvc() throws Exception {
mockMvc.perform(MockMvcRequestBuilders.post("/hi")
.contentType(MediaType.APPLICATION_JSON)
.content("{\"code\":\"ddd-d\",\"name\":\"this is name\"}")
)
.andExpect(MockMvcResultMatchers.status().isOk())
.andDo(MockMvcResultHandlers.print())
.andExpect(MockMvcResultMatchers.jsonPath("code").value(0))
.andReturn();
}
}
参考
Views: 1,426 · Posted: 2022-10-16
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
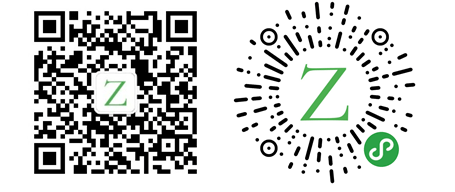
Loading...