前端 Chrome 反调试方法
Chrome JavaScript debugger About 2,935 words反调试
前端代码可以在开发者工具中看到,还可以查看网络请求的数据等。限制别人打开开发者工具或者让别人用不了开发者工具就达到反调试的目的了。
常规方法
监听是否打开开发者工具,若打开,则直接调用JavaScript
的window.close()
方法关闭网页。
方法一
监听F12
按键、监听Ctrl+Shift+I
(Windows系统)组合键、监听右键菜单。监听Ctrl+s
是为了禁止保存至本地,避免被Overrides
。
<script>
//监听F12、Ctrl+Shift+i、Ctrl+s
document.onkeydown = function (event) {
if (event.key === "F12") {
window.close();
window.location = "about:blank";
} else if (event.ctrlKey && event.shiftKey && event.key === "I") {//此处I必须大写
window.close();
window.location = "about:blank";
} else if (event.ctrlKey && event.key === "s") {//此处s必须小写
event.preventDefault();
window.close();
window.location = "about:blank";
}
};
//监听右键菜单
document.oncontextmenu = function () {
window.close();
window.location = "about:blank";
};
</script>
方法二
监听窗口大小变化。
<script>
var h = window.innerHeight, w = window.innerWidth;
window.onresize = function () {
if (h !== window.innerHeight || w !== window.innerWidth) {
window.close();
window.location = "about:blank";
}
}
</script>
方法三
利用Console.log
。
<script>
//控制台打开的时候回调方法
function consoleOpenCallback(){
window.close();
window.location = "about:blank";
return "";
}
//立即运行函数,用来检测控制台是否打开
!function () {
// 创建一个对象
let foo = /./;
// 将其打印到控制台上,实际上是一个指针
console.log(foo);
// 要在第一次打印完之后再重写toString方法
foo.toString = consoleOpenCallback;
}()
</script>
使用 debugger
第一种:constructor
<script>
function consoleOpenCallback() {
document.body.innerHTML='年轻人,不要太好奇';
window.close();
window.location = "about:blank";
}
setInterval(function () {
const before = new Date();
(function(){}).constructor("debugger")();
// debugger;
const after = new Date();
const cost = after.getTime() - before.getTime();
if (cost > 100) {
consoleOpenCallback();
}
}, 1000);
</script>
第二种:Function
<script>
setInterval(function () {
const before = new Date();
(function (a) {
return (function (a) {
return (Function('Function(arguments[0]+"' + a + '")()'))
})(a)
})('bugger')('de');
// Function('debugger')();
// debugger;
const after = new Date();
const cost = after.getTime() - before.getTime();
if (cost > 100) {
consoleOpenCallback2();
}
}, 1000);
</script>
第三方库
有大佬写了一个库专门用来判断是否打开了开发者工具,可供参考使用:
https://github.com/sindresorhus/devtools-detect
文章代码
https://github.com/fendoudebb/learning/blob/master/javascript/debugger.html
参考
Views: 6,165 · Posted: 2020-05-18
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
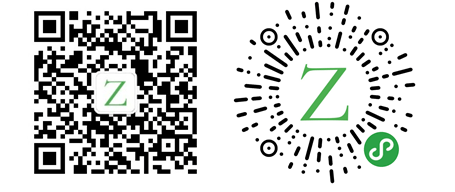
Loading...