Java 并发编程之 AtomicInteger AtomicLong
Java juc About 2,098 words说明
AtomicInteger
和AtomicLong
原子类,保证了并发时线程安全的累加操作。底层是使用CAS
原理。
AtomicInteger
原子整型类
AtomicInteger atomicInteger = new AtomicInteger();
// 获取当前的值
atomicInteger.get();
// 先获取当前的值,再加 1
atomicInteger.getAndIncrement();
// 先加1,再获取加 1 后的值
atomicInteger.incrementAndGet();
// 比较并交换
atomicInteger.compareAndSet(0, 2021);
AtomicLong
原子长整型类
AtomicLong atomicLong = new AtomicLong();
atomicLong.get();
atomicLong.getAndIncrement();
atomicLong.incrementAndGet();
atomicLong.compareAndSet(2, 2021);
小案例
public class AtomicLongDemo {
public static void main(String[] args) throws InterruptedException {
AtomicLong atomicLong = new AtomicLong();
List<Thread> threads = new ArrayList<>();
for (int i = 0; i < 10; i++) {
Thread thread = new Thread(() -> {
while (true) {
long prev = atomicLong.get();
long next = prev + 100;
boolean result = atomicLong.compareAndSet(prev, next);
if (result) {
System.out.println("success thread#" + Thread.currentThread().getName() + ", prev#" + prev + ", next#" + next);
break;
} else {
System.out.println("fail thread#" + Thread.currentThread().getName() + ", prev#" + prev + ", next#" + next);
}
}
}, "t" + i);
threads.add(thread);
}
threads.forEach(Thread::start);
for (Thread thread : threads) {
thread.join();
}
System.out.println(atomicLong.get());
}
}
输出:
success thread#t5, prev#300, next#400
success thread#t4, prev#600, next#700
success thread#t0, prev#0, next#100
success thread#t6, prev#200, next#300
fail thread#t7, prev#100, next#200
success thread#t3, prev#400, next#500
success thread#t2, prev#500, next#600
success thread#t9, prev#100, next#200
success thread#t1, prev#700, next#800
fail thread#t8, prev#600, next#700
success thread#t8, prev#900, next#1000
success thread#t7, prev#800, next#900
1000
Views: 2,082 · Posted: 2021-09-19
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
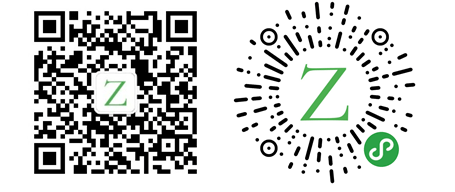
Loading...