Java 使用 MapStruct 转换对象
Java MapStruct About 4,140 words介绍
MapStruct
的作用是将类A
转换为类B
,省去了转换的setter
、getter
编码。
插件
IDEA
可安装MapStruct
插件。
添加依赖
<dependency>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct</artifactId>
<version>1.4.2.Final</version>
</dependency>
添加插件
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.5.1</version>
<configuration>
<source>11</source>
<target>11</target>
<annotationProcessorPaths>
<path>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct-processor</artifactId>
<version>1.4.2.Final</version>
</path>
</annotationProcessorPaths>
</configuration>
</plugin>
</plugins>
</build>
配合Lombok
时需添加插件lombok
。
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.5.1</version>
<configuration>
<source>11</source>
<target>11</target>
<annotationProcessorPaths>
<path>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.22</version>
</path>
<path>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct-processor</artifactId>
<version>1.4.2.Final</version>
</path>
</annotationProcessorPaths>
</configuration>
</plugin>
映射关系
类 A
@Data
public class User {
private String name;
private int age;
private String[] hobby;
private int gender;
}
类 B
@Data
public class UserDto {
private String name;
private int age;
private String[] hobby;
private String sex;
private String degree;
}
相同字段映射
User
是需要被转换的类。
UserDto
是转换后的类。
如果都是相同字段,则无需额外操作。
import org.mapstruct.Mapper;
@Mapper
public interface UserMapper {
UserMapper INSTANCE = Mappers.getMapper(UserMapper.class);
UserDto toDto(User user);
}
不同字段映射
User
中的gender
字段对应于UserDto
类的sex
。
@Mapper
public interface UserMapper {
UserMapper INSTANCE = Mappers.getMapper(UserMapper.class);
@Mapping(source = "user.gender", target = "sex")
UserDto toDto1(User user);
}
多个类
类C
@Data
public class Education {
private String degreeName;
}
将Education
中的degreeName
映射到UserDto
中的degree
。
@Mapper
public interface UserMapper {
UserMapper INSTANCE = Mappers.getMapper(UserMapper.class);
@Mapping(source = "education.degreeName", target = "degree")
UserDto toDto2(User user, Education education);
}
子对象映射
@Data
public class User {
private List<Address> addressList;
}
@Data
public class UserDto {
private List<AddressDto> addressDtoList;
}
创建子对象映射类
@Mapper
public interface AddressMapper {
AddressMapper INSTANCE = Mappers.getMapper(AddressMapper.class);
AddressDto toDto(Address address);
}
@Mapper
注解中使用uses
参数
@Mapper(uses = AddressMapper.class)
public interface UserMapper {
UserMapper INSTANCE = Mappers.getMapper(UserMapper.class);
@Mapping(source = "user.addressList", target = "addressDtoList")
UserDto toDto3(User user);
}
映射日期
实体类
@Data
public class User {
private LocalDateTime birthday;
}
@Data
public class UserDto {
private String birthdayStr;
}
Mapper
@Mapper
public interface UserMapper {
UserMapper INSTANCE = Mappers.getMapper(UserMapper.class);
@Mapping(source = "birthday", target = "birthdayStr", dateFormat = "dd/MMM/yyyy")
UserDto toDto4(User user);
}
参考
https://zhuanlan.zhihu.com/p/368731266
官网
Views: 1,445 · Posted: 2022-06-05
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
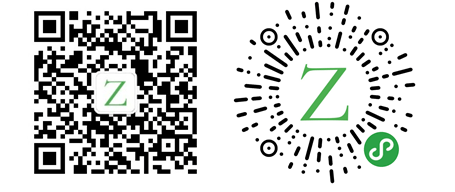
Loading...