Java JMX MemoryPoolMXBean
JMX Java About 6,683 words功能
MemoryPoolMXBean
可以获取Java
服务的内存管理器信息:
CodeCacheManager
: 代码缓存管理器Metaspace Manager
: 元数据管理器G1 Young Generation
:G1
年轻代G1 Old Generation
:G1
老年代
代码
public class MemoryPoolMXBeansTest {
public static void main(String[] args) {
List<MemoryPoolMXBean> memoryPoolMXBeans = ManagementFactory.getMemoryPoolMXBeans();
for (MemoryPoolMXBean memoryPoolMXBean : memoryPoolMXBeans) {
System.out.println("ObjectName: " + memoryPoolMXBean.getObjectName());
System.out.println("Name: " + memoryPoolMXBean.getName());
System.out.println("IsValid: " + memoryPoolMXBean.isValid());
System.out.println("MemoryManagerNames: " + Arrays.toString(memoryPoolMXBean.getMemoryManagerNames()));
System.out.println("MemoryType: " + memoryPoolMXBean.getType().name());
System.out.println("MemoryUsage: " + memoryPoolMXBean.getUsage().toString());
System.out.println("PeakUsage: " + memoryPoolMXBean.getPeakUsage());
if (memoryPoolMXBean.isUsageThresholdSupported()) {
System.out.println("UsageThreshold: " + memoryPoolMXBean.getUsageThreshold());
System.out.println("UsageThresholdCount: " + memoryPoolMXBean.getUsageThresholdCount());
System.out.println("IsUsageThresholdSupported: " + memoryPoolMXBean.isUsageThresholdSupported());
} else {
System.out.println("Usage Threshold Not Support");
}
if (memoryPoolMXBean.isCollectionUsageThresholdSupported()) {
System.out.println("CollectionUsage: " + memoryPoolMXBean.getCollectionUsage().toString());
System.out.println("CollectionUsageThreshold: " + memoryPoolMXBean.getCollectionUsageThreshold());
System.out.println("CollectionUsageThresholdCount: " + memoryPoolMXBean.getCollectionUsageThresholdCount());
System.out.println("IsCollectionUsageThresholdExceeded: " + memoryPoolMXBean.isCollectionUsageThresholdExceeded());
} else {
System.out.println("Collection Usage Not Support");
}
memoryPoolMXBean.resetPeakUsage();
System.out.println("---------------------------------");
}
}
}
输出
ObjectName: java.lang:type=MemoryPool,name=CodeHeap 'non-nmethods'
Name: CodeHeap 'non-nmethods'
IsValid: true
MemoryManagerNames: [CodeCacheManager]
MemoryType: NON_HEAP
MemoryUsage: init = 2555904(2496K) used = 1097984(1072K) committed = 2555904(2496K) max = 5849088(5712K)
PeakUsage: init = 2555904(2496K) used = 1138176(1111K) committed = 2555904(2496K) max = 5849088(5712K)
UsageThreshold: 0
UsageThresholdCount: 0
IsUsageThresholdSupported: true
Collection Usage Not Support
---------------------------------
ObjectName: java.lang:type=MemoryPool,name=Metaspace
Name: Metaspace
IsValid: true
MemoryManagerNames: [Metaspace Manager]
MemoryType: NON_HEAP
MemoryUsage: init = 0(0K) used = 7631824(7452K) committed = 8257536(8064K) max = -1(-1K)
PeakUsage: init = 0(0K) used = 7631824(7452K) committed = 8257536(8064K) max = -1(-1K)
UsageThreshold: 0
UsageThresholdCount: 0
IsUsageThresholdSupported: true
Collection Usage Not Support
---------------------------------
ObjectName: java.lang:type=MemoryPool,name=CodeHeap 'profiled nmethods'
Name: CodeHeap 'profiled nmethods'
IsValid: true
MemoryManagerNames: [CodeCacheManager]
MemoryType: NON_HEAP
MemoryUsage: init = 2555904(2496K) used = 854016(834K) committed = 2555904(2496K) max = 122896384(120016K)
PeakUsage: init = 2555904(2496K) used = 855808(835K) committed = 2555904(2496K) max = 122896384(120016K)
UsageThreshold: 0
UsageThresholdCount: 0
IsUsageThresholdSupported: true
Collection Usage Not Support
---------------------------------
ObjectName: java.lang:type=MemoryPool,name=Compressed Class Space
Name: Compressed Class Space
IsValid: true
MemoryManagerNames: [Metaspace Manager]
MemoryType: NON_HEAP
MemoryUsage: init = 0(0K) used = 733672(716K) committed = 917504(896K) max = 1073741824(1048576K)
PeakUsage: init = 0(0K) used = 733672(716K) committed = 917504(896K) max = 1073741824(1048576K)
UsageThreshold: 0
UsageThresholdCount: 0
IsUsageThresholdSupported: true
Collection Usage Not Support
---------------------------------
ObjectName: java.lang:type=MemoryPool,name=G1 Eden Space
Name: G1 Eden Space
IsValid: true
MemoryManagerNames: [G1 Old Generation, G1 Young Generation]
MemoryType: HEAP
MemoryUsage: init = 27262976(26624K) used = 4194304(4096K) committed = 27262976(26624K) max = -1(-1K)
PeakUsage: init = 27262976(26624K) used = 4194304(4096K) committed = 27262976(26624K) max = -1(-1K)
Usage Threshold Not Support
CollectionUsage: init = 27262976(26624K) used = 0(0K) committed = 0(0K) max = -1(-1K)
CollectionUsageThreshold: 0
CollectionUsageThresholdCount: 0
IsCollectionUsageThresholdExceeded: false
---------------------------------
ObjectName: java.lang:type=MemoryPool,name=G1 Old Gen
Name: G1 Old Gen
IsValid: true
MemoryManagerNames: [G1 Old Generation, G1 Young Generation]
MemoryType: HEAP
MemoryUsage: init = 509607936(497664K) used = 0(0K) committed = 509607936(497664K) max = 8589934592(8388608K)
PeakUsage: init = 509607936(497664K) used = 0(0K) committed = 509607936(497664K) max = 8589934592(8388608K)
UsageThreshold: 0
UsageThresholdCount: 0
IsUsageThresholdSupported: true
CollectionUsage: init = 509607936(497664K) used = 0(0K) committed = 0(0K) max = 8589934592(8388608K)
CollectionUsageThreshold: 0
CollectionUsageThresholdCount: 0
IsCollectionUsageThresholdExceeded: false
---------------------------------
ObjectName: java.lang:type=MemoryPool,name=G1 Survivor Space
Name: G1 Survivor Space
IsValid: true
MemoryManagerNames: [G1 Old Generation, G1 Young Generation]
MemoryType: HEAP
MemoryUsage: init = 0(0K) used = 0(0K) committed = 0(0K) max = -1(-1K)
PeakUsage: init = 0(0K) used = 0(0K) committed = 0(0K) max = -1(-1K)
Usage Threshold Not Support
CollectionUsage: init = 0(0K) used = 0(0K) committed = 0(0K) max = -1(-1K)
CollectionUsageThreshold: 0
CollectionUsageThresholdCount: 0
IsCollectionUsageThresholdExceeded: false
---------------------------------
ObjectName: java.lang:type=MemoryPool,name=CodeHeap 'non-profiled nmethods'
Name: CodeHeap 'non-profiled nmethods'
IsValid: true
MemoryManagerNames: [CodeCacheManager]
MemoryType: NON_HEAP
MemoryUsage: init = 2555904(2496K) used = 174976(170K) committed = 2555904(2496K) max = 122912768(120032K)
PeakUsage: init = 2555904(2496K) used = 174976(170K) committed = 2555904(2496K) max = 122912768(120032K)
UsageThreshold: 0
UsageThresholdCount: 0
IsUsageThresholdSupported: true
Collection Usage Not Support
---------------------------------
Views: 236 · Posted: 2024-01-08
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
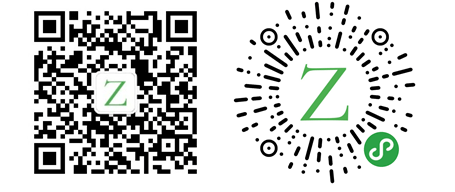
Loading...